Python internal API#
neophile Package#
The neophile service.
Variables#
The application version string (PEP 440 / SemVer compatible). |
neophile.analysis.base Module#
Base class for an analysis step.
Classes#
Base class for an analysis step. |
Class Inheritance Diagram#

neophile.analysis.pre_commit Module#
Analysis of a repository for needed pre-commit hook updates.
Classes#
|
Analyze a tree for needed pre-commit hook updates. |
Class Inheritance Diagram#

neophile.analysis.python Module#
Analysis of a repository for needed Python updates.
Classes#
Analyze a tree for needed Python frozen dependency updates. |
Class Inheritance Diagram#

neophile.cli Module#
Command-line interface.
neophile.config Module#
Configuration for neophile.
Classes#
Configuration for neophile. |
Class Inheritance Diagram#

neophile.exceptions Module#
Exceptions for neophile.
Classes#
The specified dependency was not found to update. |
|
Pushing a branch to GitHub failed. |
|
The repository contains uncommitted changes. |
Class Inheritance Diagram#
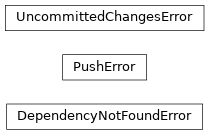
neophile.factory Module#
Factory for neophile components.
Classes#
|
Factory to create neophile components. |
Class Inheritance Diagram#

neophile.inventory.github Module#
Inventory of available GitHub tags.
Classes#
|
Inventory available tags of a GitHub repository. |
Class Inheritance Diagram#
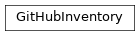
neophile.inventory.version Module#
Version representation for inventories.
Classes#
|
Represents a version string using |
Abstract base class for versions. |
|
|
Represents a semantic version string. |
Class Inheritance Diagram#
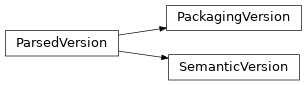
neophile.models.dependencies Module#
Representations of dependencies.
Classes#
|
Base class for a dependency returned by a scanner. |
|
Represents a single Helm dependency. |
|
Represents a single Kustomize dependency. |
|
Represents a single pre-commit dependency. |
Class Inheritance Diagram#
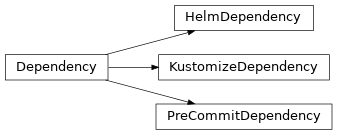
neophile.pr Module#
Construct a GitHub PR for a set of changes.
Classes#
|
A Git commit message. |
|
An individual GitHub repository. |
|
Create GitHub pull requests. |
Class Inheritance Diagram#
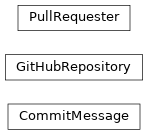
neophile.processor Module#
Process a set of repositories for updates.
Classes#
|
Process a set of repositories for updates. |
Class Inheritance Diagram#

neophile.repository Module#
Wrapper around a Git repository.
Classes#
|
Wrapper around a Git repository to add some convenience functions. |
Class Inheritance Diagram#
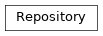
neophile.scanner.base Module#
Base class for dependency scanners.
Classes#
Base class for dependency scanners. |
Class Inheritance Diagram#

neophile.scanner.pre_commit Module#
pre-commit hook dependency scanning.
Classes#
Scan a source tree for pre-commit hook version references. |
Class Inheritance Diagram#

neophile.update.base Module#
Base class for dependency updates.
Notes
This is a bit more complicated than it should have to be to work around python/mypy#5374. The mixins avoid the conflict between an abstract base class and a dataclass in mypy’s understanding of the Python type system.
Classes#
Add the abstract methods for an update. |
|
|
Base class for a needed dependency version update. |
|
Add the base data elements for |
Class Inheritance Diagram#
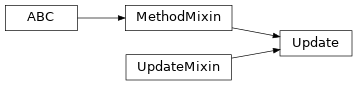
neophile.update.pre_commit Module#
pre-commit dependency update.
Classes#
|
An update to a Helm chart dependency. |
Class Inheritance Diagram#

neophile.update.python Module#
Python frozen dependency update.
Classes#
|
An update to Python frozen dependencies. |
Class Inheritance Diagram#
